This may not be the only or even best way of preventing backdated entries in myEvolv but this method worked for me in a recent configuration.
Scenario
We wanted our prescribers to be able to provide a Script for Therapy Services to children in our preschool services program from myEvolv. Scripts for Service must have a start date on them and that start date needs to be no earlier than the date indicated on the child’s IEP but cannot but previous to the date that the script is being signed.
Further, an administrative assistant was going to be setting up the scripts in myEvolv and then the prescribers were going to be reviewing and e-signing them. We could imagine a scenario where the administrative assistant put a date, for example (9/1) on the script, believing that the prescriber would e-sign it on or before that date but the prescriber does not get into myEvolv to sign it until after that date has passed and then the script gets e-signed as though it were backdated. We needed a way to prevent the start date from ever being previous to the approved date while also allowing the start date to be in the future.
Form Design
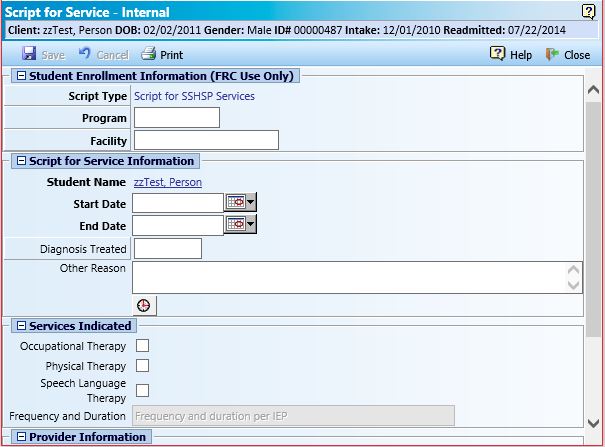
The actual_date
field was not suitable for use as the script start date since we needed to be able have multiple scripts that start on the same day for an individual. If I set the actual date as ‘Date Only’ Display Type Code, then two scripts in the same day would overlap at 00:00 AM. In this case I ended up creating a new database field for my Start Date and kept actual date on the form, receiving todaysDate()
as the default value. This more or less prevents the service overlap problem. My new database field is named udf_rx_4_svc_start
and is Date Only.
The end date on the script is using the expiration_date
column and is also using the Display Type Code “Date Only”.
Before Save Code
The first task to accomplish is to prevent a user from saving the form with a backdate on it. For this I used the Before Save code property on the form. This code executes every time that the form is saved BUT because of the algorithm myEvolv uses to save form data, the udf_rx_4_svc_start
column in the database will only be updated if the form field has been flagged as being modified. This is something that I was not able to figure out how to do in the Before Save code yet and is largely responsible for what feels like a more-complex-than-is-strictly-necessary-solution — more on that later.
First step is to get the value that is on the form field and turn it into a JavaScript date object.
var start = new Date(getFormElement('udf_rx_4_svc_start'));
Second step is to get today’s date and turn it into a JavaScript date object. todaysDate()
is a myEvolv form function that you can use to get today’s date in a format suitable for placing in a myEvolv form field.
var today = new Date (todaysDate());
With that accomplished, we can compare the two date objects to see if the date on the form is previous to today’s date and if so, set the form field’s value to today’s date.
if(start <= today){{setFormElement('udf_rx_4_svc_start', todaysDate())}};
Note that the {
and }
brackets are doubled up in the conditional statement. This will prevent the “Not a Valid XSLT or XPath Function” error previously discussed here.
With this code in place, when a new Script for Service is entered or a draft Script for Service has it’s udf_rx_4_svc_start
field modified, myEvolv will check to make sure that the start date is not not in the past and if it is, update the start date to today’s date. If the date is today’s date or a future date, it is left as it is.
Full Code Block
var start = new Date(getFormElement('udf_rx_4_svc_start'));
var today = new Date (todaysDate());
if(start <= today){{setFormElement('udf_rx_4_svc_start', todaysDate())}};
On Load Script
Just the Before Save Code alone would not be enough to prevent backdating. We still have the possibility of someone simply opening the draft and e-signing the form as in our scenario where the prescriber gets into myEvolv to e-sign scripts later than intended. Even if the form is edited in some way, if the Start Date is not touched, the Before Save code will execute but the column will not be flagged as modified and myEvolv will ignore it when updating the database. My approach here was to try to update the form as it loads upon viewing or editing so that myEvolv would see the column as modified when saving. I was unable to get the toolbar containing the ‘Save’ button to recongize the change, however, I was able to still get the desired effect another way.
The first thing to keep in mind with the On Load Script is that the form DOM has not loaded yet when this code runs. You can not therefore target the form field with JavaScript, update its value and focus/blur to make myEvolv notice a change to the value as we can with the On Click and On Change Scripts. Instead, you have to manipulate the Record XML Data before it gets loaded into the form.
The first step here is to determine whether the script had been signed. If it has been signed, it will have a non-null date_locked
value. We check for this by using the myEvolv form function getDataValue()
and then seeing if the form is being opened for viewing or editing.
var locked = getDataValue('event_log', 'event_log_id', keyValue, 'date_locked');
if(locked == '' && formXML.documentElement.getAttribute('formAction') == 'EDIT'){{
...
}}
This code gets the value of date_locked
from the event_log
table where the event_log_id
is equal to keyValue which is a variable equal to the event log id of the current event you are looking at.
Then the code checks to see if there is a locked_date. If the locked date is blank AND the form is opened for editing, then it will execute the rest of the code. So basically, only perform a date manipulation if we are still working with a draft. If you leave this piece out then every time you look at a completed script for service in the future, myEvolv will overwrite the form field value for start date to be today’s date and you will be scratching your head.
Inside the ellipses we start the date manipulation. First we load the node for udf_rx_4_svc_start
from the XML Record so that we can manipulate it.
node = formXML.documentElement.selectNodes("form_group/form_item [@column_name = 'udf_rx_4_svc_start']");
node = node[0];
This code returns an Array of all of the nodes in the XML documents with the column_name “udf_rx_svc_start”. There is only one but it is in an array so we load it into our variable directly on the next line.
Then we get the start date value and turn it into a JavaScript date object.
var start = new Date(node.text);
Then we do the same for today’s date.
var today = new Date(todaysDate());
Now we check to see if start date is before today’s date and if so, we will update the text of the node to be today’s date.
if(start < today){{
node.text = todaysDate();
node.setAttribute('modified', 'true');
...
}}
I also set the “modified” attribute to ‘true’ for this node. I don’t know if that makes a difference in this setup but I am including it since that is how my code is and it works.
If you leave the code as it is like this, when the form loads, if the start date is previous to today’s date, the value on the form will have been updated to today’s date AND the form field will be maroon like it is when a form field is changed. However, you will also notice that the “Save” button is nevertheless disabled and a user would be able to e-sign the service without being forced to hit save. And apparently, even though a “saving…” dialog pops up upon e-signing, the form is not saved. I tried a bunch of things to try to get the save button to enable and the e-sign button to disable but it is difficult since the form DOM is not loaded at the time that the On Load script runs. In the end, I decided to just call the save form function directly.
So where the ellipses above are, I put
SaveForm2();
The end result here is that when the form is opened for editing, if the start date is before today’s date, myEvolv will update the start date to be today’s date and then save. The users will see the form start to open and then abruptly close if the conditions are met. When they reopen it, they will find that today’s date has been updated to today’s date and they are free to e-sign.
Full Code Block
var locked = getDataValue('event_log', 'event_log_id', keyValue, 'date_locked');
if(locked == '' && formXML.documentElement.getAttribute('formAction') == 'EDIT'){{
node = formXML.documentElement.selectNodes("form_group/form_item [@column_name = 'udf_rx_4_svc_start']");
node = node[0];
var start = new Date(node.text);
var today = new Date(todaysDate());
if(start < today){{
node.text = todaysDate();
node.setAttribute('modified', 'true');
SaveForm2();
}}
}}
Bonus: After Save Code
Since the form is going to be auto-saving itself, I also added a snippet of code to the After Save Code that keeps the form from closing after save:
window.closeAfterSave = false;